Loops in C
Loops in C language are used to repeatedly execute a block of code as long as a certain condition is met. This allows for more efficient and concise programming, especially in cases where the same code needs to be executed multiple times with only slight variations. The three types of loops in C are the for
loop, while
loop, and do-while
loop, each with their own specific use cases. Common applications of loops in C include iterating over arrays, processing input data, and implementing algorithms that require repeated execution.
For Loop
for loop is used to repeat code when given condition become true. Loop has three section initializer, condition and update. initializer will execute only once, then every iteration condition and update.
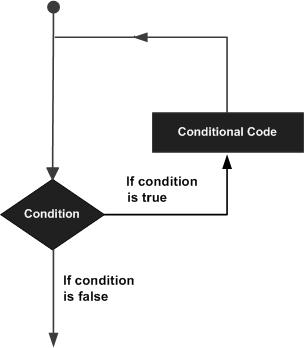
For Loop Syntax:
for (initilize; condition; update)
{
/* code */
}
Example:
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char const *argv[])
{
int i;
for (i = 0; i < 10; i++)
{
printf(" %d",i);
}
return 0;
}
While Loop
while
loop is same as for loop but, it is mostly used when iteration time is unknown. Using this loop we have to specify the terminating condition otherwise loop become infinite and it is dangerous for computer.
While loop Syntax:
while (/* condition */)
{
/* code */
}
while loop Example:
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char const *argv[])
{
int i =0;
while (i <= 50)
{
printf(" %d",i);
i = i+5;
}
return 0;
}
Do-While() Loop
do-while
loop is used same as while loop , but in do while loop first execute the code then check the condition, this concept is used.
do while loop Syntax:
do
{
/* code */
} while (/* condition */);
Examples:
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char const *argv[])
{
int i =0;
do
{
i = i+5;
printf(" %d",i);
}while (i <= 50);
return 0;
}
NOTE: In Every loop you must have specify terminating condition otherwise loop goes infinite and it is bad for system.